[ODOP] 68일차 - AntPathMatcher - 테스트
ODOP(One Day One Posting) 68일차. AntPathMatcher 의 테스트와 코드 분석을 통해 match, matchStart, extractUriTemplateVariables 함수의 사용 방법을 정리했습니다
![[ODOP] 68일차 - AntPathMatcher - 테스트](/content/images/size/w1200/2023/08/-----2023-08-01----10.29.26-3.png)
💡
ODOP 는 One Day One Post 의 약자로 이후 ODOP 형태의 블로깅에는 해당 문구를 포함할 예정이다
doMatch 함수
protected boolean doMatch(String pattern, @Nullable String path, boolean fullMatch, @Nullable Map<String, String> uriTemplateVariables)
실제로 주어진 경로를 주어진 패턴과 일치시킵니다.
pattern: 일치 시킬 패턴
path: 패턴이 일치하는지 테스트 할 path
fullMatch: 전체 패턴 일치가 필요한지 여부(지정된 기본 경로만 일치하면 패턴 일치로 충분함)
uriTemplateVariables: (주석에는 별다른 설명이 없다)
내부적으로 doMatch 함수를 이용하는 로직은 세개가 존재한다
- public boolean matchStart(String pattern, String path)
- public boolean match(String pattern, String path)
- public Map<String, String> extractUriTemplateVariables(String pattern, String path)
match 함수
먼저 테스트 해 볼 것은 match 함수이다
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import org.springframework.util.AntPathMatcher;
public class AntPathMatcherTest {
@Test
void matchTest() {
AntPathMatcher antPathMatcher = new AntPathMatcher();
String requestPath = "/api/users/10000";
boolean match = antPathMatcher.match("/api/users/{userId}", requestPath);
assertTrue(match); // 결과는 true
}
}
어차피 path 의 구분자는 기본 구분자( /
) 가 들어갈테니 따로 추가해주지는 않았다.
기본적인 경로 매치에는 정말 간단하게 성공하는 모습을 보인다
matchStart 함수
그럼 matchStart 는 무엇일까?
메서드에 나와있는 그대로 요청된 path 의 시작 경로부터 검색을 시작한다
@DisplayName("AntPathMatcher")
public class AntPathMatcherTest{
@Test
void matchStartTest01() {
AntPathMatcher antPathMatcher = new AntPathMatcher();
String requestPath = "/api/users/10000";
boolean match = antPathMatcher.matchStart("/api/users/{userId}", requestPath);
assertTrue(match);
}
@Test
void matchStartTest02() {
AntPathMatcher antPathMatcher = new AntPathMatcher();
String requestPath = "/api/users";
boolean match = antPathMatcher.matchStart("/api/users/{userId}", requestPath);
assertTrue(match);
}
@Test
void matchStartTest03() {
AntPathMatcher antPathMatcher = new AntPathMatcher();
String requestPath = "/api";
boolean match = antPathMatcher.matchStart("/api/users/{userId}", requestPath);
assertTrue(match);
}
}
세 테스트의 결과는 모두 true 가 나오게된다
시작하는 문자열부터 검색하여 처리된다
extractUriTemplateVariables 함수
그럼 이 녀석은 무엇일까?
시그니쳐를 확인해보자
public Map<String, String> extractUriTemplateVariables(String pattern, String path)
해당 메서드는 주어진 path 에서 동적인 부분과 정적인 부분을 검사하여 동적인 부분에 대한 매치를 수행하고, 해당 값을 리턴하는 역할을 수행한다
ant-style 의 path 에서 우리는 이러한 경로를 사용 할 수 있다.
/api/theaters/{theaterName}/movies/{movieId}/seats/{seatCode}
PathVariable 은 path 경로 사이에 특정한 키워드를 통해 동적인 값의 이름과 타입을 지정하고 추출하는 용도로 사용된다
이 함수는 이러한 매핑 값들을 Map 형태로 리턴 하는 함수이다
아래는 테스트를 위해 작성한 코드와 결과이다
import static org.junit.jupiter.api.Assertions.*;
import java.util.Map;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
import org.springframework.util.AntPathMatcher;
@DisplayName("AntPathMatcher")
public class AntPathMatcherTest {
@Test
void extractUriTemplateVariables() {
AntPathMatcher antPathMatcher = new AntPathMatcher();
String requestPath = "/api/theaters/test_theaters/movies/1000/seats/a-1";
Map<String, String> pathVariables = antPathMatcher.extractUriTemplateVariables(
"/api/theaters/{theaterName}/movies/{movieId}/seats/{seatCode}",
requestPath);
assertEquals("test_theaters", pathVariables.get("theaterName"));
assertEquals("1000", pathVariables.get("movieId"));
assertEquals("a-1", pathVariables.get("seatCode"));
}
}
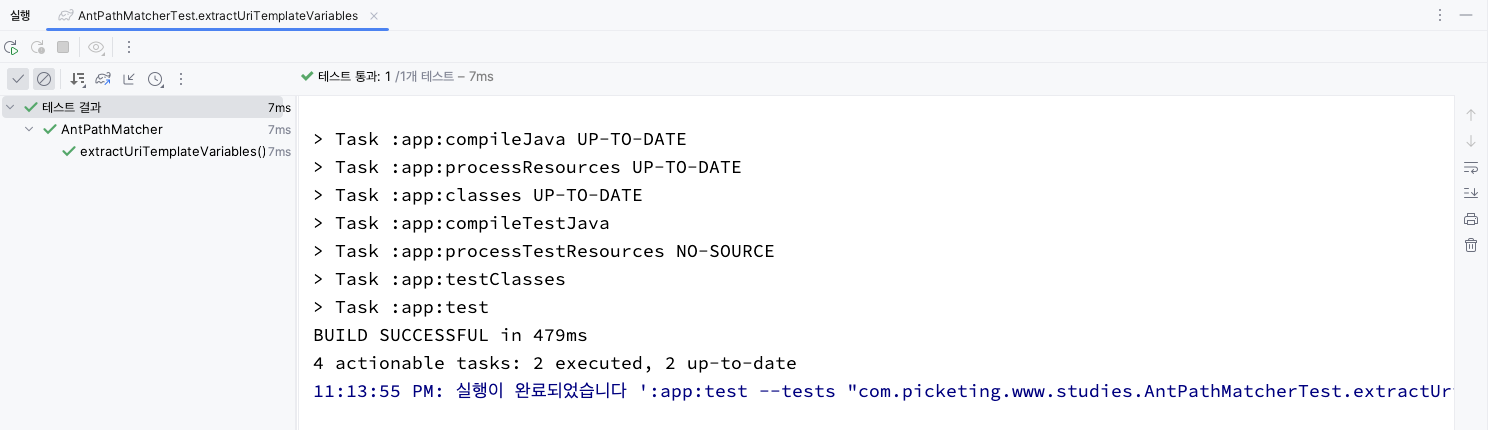